Currently there is no direct way to set a fixed filename for any of the output targets inside the Icetips Previewer, but you can easily do this on the report itself, rather than passing the name to the previewer and do it there. In fact this would probably be the preferred method since the report probably should determine the name and location of the resulting filename.
You need to put code into a single embed where you set the filename:
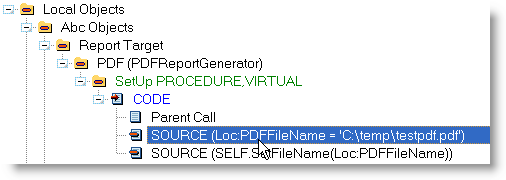
All the code that you need is just a call to the SELF.SetFileName() method. Please note that each output target has it's own Setup method so if you have multiple output target templates active you will need to do this for all of them. You can pass the filename as a string, variable or an empty string if you want it to open the file dialog in the Previewer when you print to PDF.
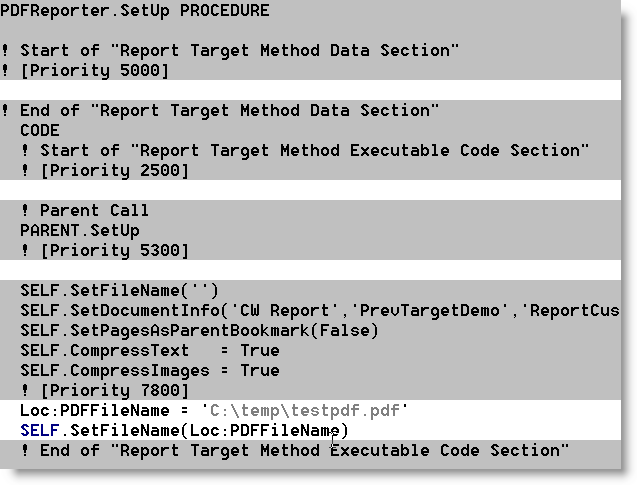
In this case you can use:
This triggers the FileDialog to open in Previewer when you click on the PDF button.
SELF.SetFileName('myfile.pdf')
This writes the PDF file as "myfile.pdf" to the current folder.
SELF.SetFileName('C:\temp\myfile.pdf')
This writes the PDF file to specific folder, which is probably not what you want to do!
SELF.SetFileName(LOC:PDFFileName)
In this case a variable is used. You can prime the variable anywhere before the PDFReporter.SetUp method is called, or you can do it right before the SELF.SetFileName. Note that the PDFREporter.Setup method is not actually called until the report is in the Previewer so if you use a Global, Threaded variable, you can set this in the Previewer. Since the previewer and the report will always be on the same thread, you can just set this variable to be threaded and then there are not threading issues to deal with.
Here is an example of how this can be implemented. On the report I have changed the code to:
SELF.SetFileName(Glo:PDFFileName)
The global variable is a CString(2049) global variable with the "THREAD" attribute. To do that simply check the "THREAD" checkbox on the "Attributes" tab when you create the global variable - doesn't make a difference if you do it in the Global Data or in the Dictionary.
In the Previewer, all that is needed now is to set the global variable. Normally I would think that you would want to set the filename on the report, but this gives you an idea how to do it on the Previewer also. I have added an instance of our ITShellClass from the Icetips Utilities to the previewer procedure - in the "Local Data" embed I added:
Now in the "Local Objects | Icetips Previewer | Clarion 6 Save Buttons | Before Save Dialog"

I have added this code:
If GQ.GName = 'PDF'
Glo:PDFFileName = ITS.GetSpecialFolder(IT_CSIDL_PERSONAL) &|
'\MyPDF.pdf'
!! Glo:PDFFileName must be be used in the report's
!! PDFREporter.Setup.
If Not FileDialog('Select PDF to save',|
Glo:PDFFileName,|
'*.pdf|*.pdf',|
FILE:SAVE+FILE:KEEPDIR+FILE:LONGNAME+FILE:ADDEXTENSION)
Message('No PDF File selected, aborting PDF save',|
'PDF Saving aborted',ICON:Hand)
Exit
End
End
Note the "GQ.GName = 'PDF'". This allows you to just do the PDF if you want the default filename handling for other options. The GQ contains all the target names, such as "PDF", "HTML" etc. At the top of the routine that handles the targets in the Previewer, the correct item is selected from the GQ queue so throughout the routine you can use GQ.GName to determine what is the active target being printed to.
If you find new ways to use this, I would certainly appreciate if you would be kind enough to add a comment to this article so I and others can benefit:)
Arnor Baldvinsson
